Resizing
Grafika support 5 ways of resizing an image: fit, fill, exact, exactWidth, and exactHeight.
Opening an Image
To open an existing image, all you need is to create an editor instance and call the open method:
require_once 'path/to/grafika/src/autoloader.php'; // Automatically load our needed classes
use Grafika\Grafika; // Import package
// Create editor
$editor = Grafika::createEditor();
// Open jpeg image and store it in $image variable
$editor->open( $image, "images/sample-jpeg.jpg" );
Resize Fit
Resize Fit will fit the image within the given dimension:
$editor->open( $image1, "images/sample.jpg" ); // Open jpeg image for editing
$editor->resizeFit( $image1, 200, 200 ); // Fit an image to 200 x 200 box
$editor->save( $image1, "images/testResizeFit.jpg"); // Save the image as jpeg
$editor->open( $image2, "images/portrait.jpg" ); // Open another image for editing
$editor->resizeFit( $image2, 200, 200 ); // Fit an image to 200 x 200 box
$editor->save( $image2, "images/testResizeFitPortrait.jpg"); // Save the image as jpeg
The gray box shows the 200x200 dimension that the images fit in.
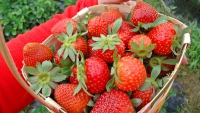
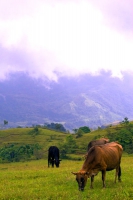
Resize Exact
Resize Exact will use the exact dimensions given, ignoring the aspect ratio. Here images appear squashed. It is useful if you want to force exact dimensions:
$editor->open( $image1, "images/sample.jpg" );
$editor->resizeExact( $image1, 200, 200 );
$editor->save( $image1, "images/testResizeExact.jpg");
$editor->open( $image2, "images/portrait.jpg" );
$editor->resizeExact( $image2, 200, 200 );
$editor->save( $image2, "images/testResizeExactPortrait.jpg");
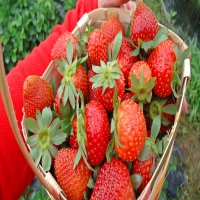
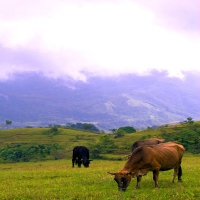
Resize Fill
Resize Fill will fill the entire dimension given. Excess parts are cropped:
$editor->open( $image1, "images/sample.jpg" );
$editor->resizeFill( $image1, 200, 200 );
$editor->save( $image1, "images/testResizeFill.jpg");
$editor->open( $image2, "images/portrait.jpg" );
$editor->resizeFill( $image2, 200, 200 );
$editor->save( $image2, "images/testResizeFillPortrait.jpg");
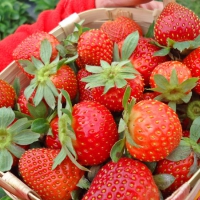
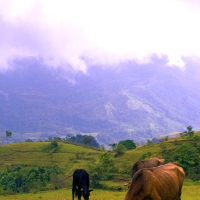
Resize Exact Width
With resizeExactWidth, the height is auto calculated. Useful if you want column of images to be exactly the same width:
$editor->open( $image1, "images/sample.jpg" );
$editor->resizeExactWidth( $image1, 100 );
$editor->save( $image1, "images/testResizeExactWidth.jpg");
$editor->open( $image2, "images/portrait.jpg" );
$editor->resizeExactWidth( $image2, 100 );
$editor->save( $image2, "images/testResizeExactWidthPortrait.jpg");
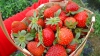
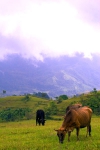
Resize Exact Height
With resizeExactHeight, the width is auto calculated. Useful if you want row of images to be exactly the same height:
$editor->open( $image1, "images/sample.jpg" );
$editor->resizeExactHeight( $image1, 100 );
$editor->save( $image1, "images/testResizeExactHeight.jpg");
$editor->open( $image2, "images/portrait.jpg" );
$editor->resizeExactHeight( $image2, 100 );
$editor->save( $image2, "images/testResizeExactHeightPortrait.jpg");
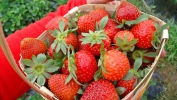
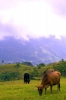